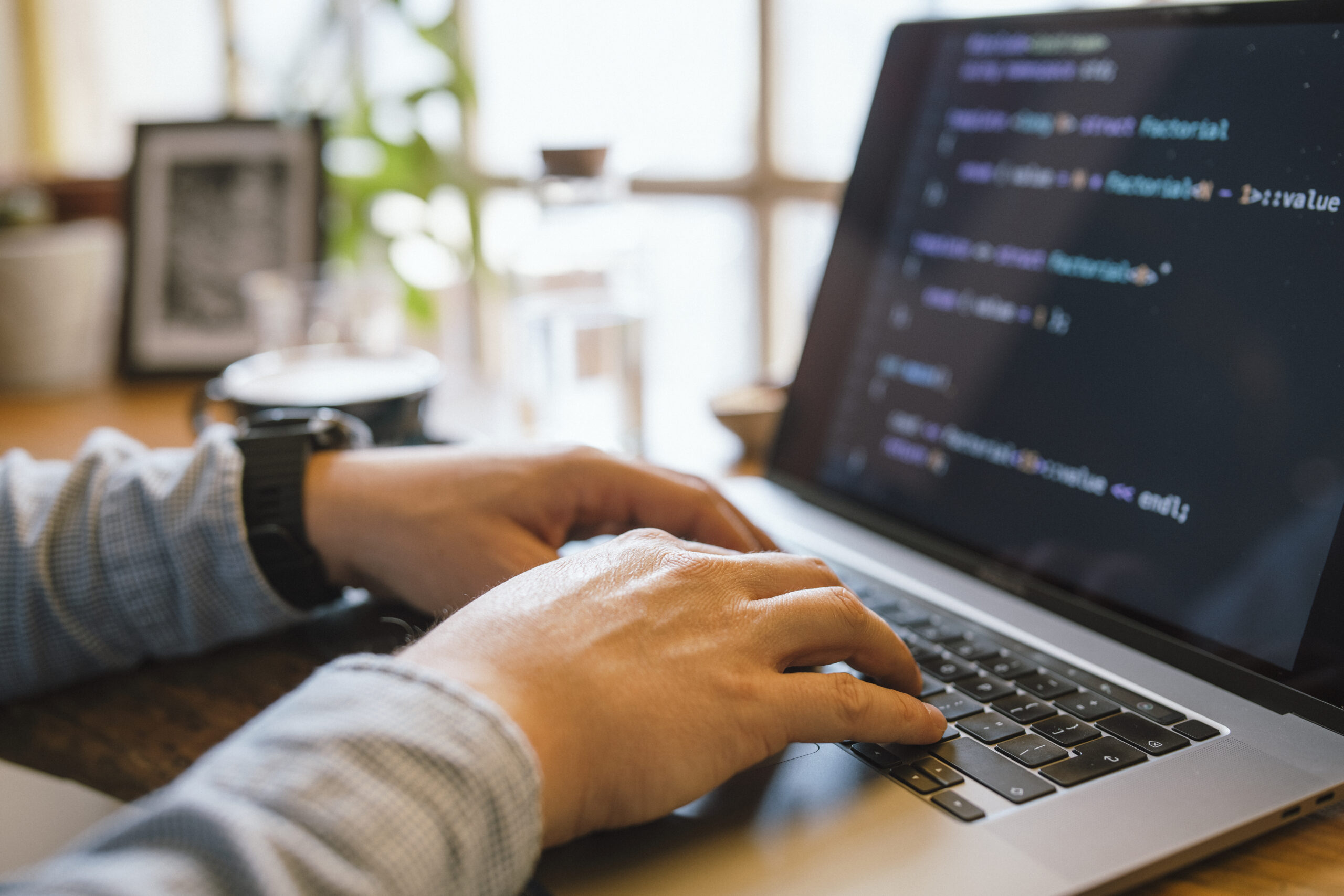
Debugging is One of the more vital — nonetheless often disregarded — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about knowing how and why factors go Erroneous, and Discovering to Imagine methodically to unravel problems efficiently. Whether or not you're a newbie or even a seasoned developer, sharpening your debugging expertise can preserve hrs of disappointment and drastically boost your productiveness. Listed below are numerous methods to assist developers amount up their debugging activity by me, Gustavo Woltmann.
Grasp Your Equipment
One of several quickest methods developers can elevate their debugging skills is by mastering the applications they use on a daily basis. Even though composing code is a single A part of development, recognizing tips on how to interact with it proficiently during execution is Similarly critical. Modern day advancement environments occur equipped with impressive debugging abilities — but numerous builders only scratch the surface area of what these resources can perform.
Acquire, by way of example, an Integrated Development Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These applications permit you to set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in some cases modify code within the fly. When utilized correctly, they Permit you to observe accurately how your code behaves through execution, which can be a must have for tracking down elusive bugs.
Browser developer tools, which include Chrome DevTools, are indispensable for front-end builders. They let you inspect the DOM, keep track of community requests, watch actual-time overall performance metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can flip disheartening UI difficulties into manageable responsibilities.
For backend or method-stage developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB offer deep Management over managing procedures and memory administration. Studying these equipment might have a steeper Discovering curve but pays off when debugging efficiency issues, memory leaks, or segmentation faults.
Over and above your IDE or debugger, turn out to be snug with Edition Regulate systems like Git to grasp code record, locate the precise instant bugs had been released, and isolate problematic changes.
Eventually, mastering your applications indicates heading past default configurations and shortcuts — it’s about creating an intimate familiarity with your improvement setting so that when concerns occur, you’re not shed at midnight. The higher you already know your instruments, the greater time you can invest solving the actual dilemma in lieu of fumbling through the process.
Reproduce the issue
Just about the most vital — and often ignored — measures in powerful debugging is reproducing the situation. Ahead of leaping into your code or building guesses, builders need to have to create a constant environment or state of affairs the place the bug reliably appears. With out reproducibility, fixing a bug becomes a activity of opportunity, typically leading to squandered time and fragile code improvements.
Step one in reproducing a problem is accumulating just as much context as you can. Inquire concerns like: What actions led to The difficulty? Which surroundings was it in — improvement, staging, or production? Are there any logs, screenshots, or mistake messages? The more detail you have got, the simpler it becomes to isolate the precise situations under which the bug takes place.
As soon as you’ve collected more than enough data, attempt to recreate the problem in your neighborhood atmosphere. This may suggest inputting a similar info, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic exams that replicate the sting instances or condition transitions associated. These exams not simply assist expose the situation but also avert regressions Down the road.
At times, The problem may be surroundings-unique — it might transpire only on specific operating devices, browsers, or below unique configurations. Utilizing applications like virtual machines, containerization (e.g., Docker), or cross-browser tests platforms could be instrumental in replicating these types of bugs.
Reproducing the issue isn’t merely a step — it’s a state of mind. It involves patience, observation, plus a methodical solution. But after you can continually recreate the bug, you might be currently halfway to repairing it. By using a reproducible circumstance, You may use your debugging applications more successfully, examination likely fixes safely and securely, and converse additional Plainly with the group or end users. It turns an summary grievance right into a concrete problem — and that’s in which developers thrive.
Go through and Realize the Error Messages
Error messages are often the most valuable clues a developer has when some thing goes Incorrect. Rather than seeing them as frustrating interruptions, builders should really study to take care of mistake messages as direct communications in the system. They normally inform you what exactly occurred, in which it occurred, and from time to time even why it took place — if you understand how to interpret them.
Get started by examining the information meticulously and in complete. Many builders, specially when underneath time stress, glance at the main line and quickly commence making assumptions. But further while in the error stack or logs may possibly lie the correct root bring about. Don’t just duplicate and paste error messages into search engines like yahoo — study and have an understanding of them initially.
Break the mistake down into elements. Can it be a syntax error, a runtime exception, or a logic mistake? Will it level to a specific file and line range? What module or purpose triggered it? These issues can guidebook your investigation and level you towards the responsible code.
It’s also handy to comprehend the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable patterns, and Finding out to recognize these can greatly quicken your debugging system.
Some mistakes are imprecise or generic, and in People instances, it’s important to examine the context through which the mistake occurred. Check out related log entries, input values, and up to date changes from the codebase.
Don’t overlook compiler or linter warnings both. These frequently precede more substantial challenges and provide hints about probable bugs.
Finally, error messages will not be your enemies—they’re your guides. Learning to interpret them appropriately turns chaos into clarity, serving to you pinpoint challenges faster, minimize debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is Probably the most effective instruments inside of a developer’s debugging toolkit. When used successfully, it provides actual-time insights into how an application behaves, serving to you fully grasp what’s going on underneath the hood without having to pause execution or move in the code line by line.
A good logging technique begins with being aware of what to log and at what stage. Popular logging levels consist of DEBUG, INFO, Alert, Mistake, and Lethal. Use DEBUG for specific diagnostic facts all through enhancement, Facts for normal functions (like profitable start off-ups), WARN for potential challenges that don’t crack the appliance, ERROR for actual complications, and Deadly once the method can’t go on.
Prevent flooding your logs with extreme or irrelevant facts. Excessive logging can obscure essential messages and decelerate your process. Give attention to important situations, condition adjustments, enter/output values, and significant selection details with your code.
Format your log messages Plainly and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace challenges in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what problems are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re especially worthwhile in production environments the place stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Ultimately, sensible logging is about equilibrium and clarity. Having a properly-thought-out logging strategy, you could reduce the time it will require to identify problems, achieve further visibility into your applications, and Enhance the Over-all maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not just a complex undertaking—it's a type of investigation. To properly detect and fix bugs, developers have to tactic the procedure like a detective solving a mystery. This frame of mind allows stop working complicated concerns into manageable areas and observe clues logically to uncover the root trigger.
Commence by collecting proof. Consider the indications of the problem: error messages, incorrect output, or efficiency concerns. Similar to a detective surveys a criminal offense scene, acquire as much appropriate data as you can with out jumping to conclusions. Use logs, test cases, and user experiences to piece together a transparent picture of what’s happening.
Up coming, sort hypotheses. Inquire oneself: What may be triggering this conduct? Have any adjustments recently been made on the codebase? Has this situation transpired prior to under similar circumstances? The intention is to narrow down options and determine likely culprits.
Then, examination your theories systematically. Seek to recreate the condition in a controlled setting. When you suspect a certain perform or part, isolate it and verify if The difficulty persists. Similar to a detective conducting interviews, check with your code inquiries and Allow the outcomes direct you closer to the truth.
Pay near focus to modest specifics. Bugs frequently cover in the minimum envisioned locations—just like a lacking semicolon, an off-by-1 mistake, or even a race situation. Be extensive and patient, resisting the urge to patch the issue without the need of totally understanding it. Short term fixes may cover the actual dilemma, just for it to resurface later.
And lastly, maintain notes on what you tried out and learned. Just as detectives log their investigations, documenting your debugging system can more info conserve time for long run problems and enable Other people recognize your reasoning.
By thinking like a detective, developers can sharpen their analytical techniques, approach troubles methodically, and come to be more effective at uncovering hidden challenges in complicated devices.
Write Tests
Composing assessments is among the most effective approaches to transform your debugging skills and General growth effectiveness. Checks don't just assist catch bugs early but in addition function a security Web that offers you confidence when creating modifications to the codebase. A very well-analyzed software is much easier to debug because it allows you to pinpoint specifically in which and when a difficulty happens.
Begin with device checks, which concentrate on person functions or modules. These little, isolated exams can speedily reveal no matter if a selected bit of logic is Doing the job as expected. Each time a examination fails, you quickly know the place to seem, appreciably minimizing enough time expended debugging. Unit exams are Specially valuable for catching regression bugs—troubles that reappear soon after Formerly becoming set.
Subsequent, integrate integration checks and conclusion-to-conclusion assessments into your workflow. These aid make certain that different parts of your software operate collectively smoothly. They’re particularly handy for catching bugs that arise in sophisticated methods with multiple parts or companies interacting. If one thing breaks, your tests can inform you which Portion of the pipeline unsuccessful and beneath what circumstances.
Crafting exams also forces you to definitely Assume critically about your code. To check a function adequately, you will need to understand its inputs, anticipated outputs, and edge cases. This standard of knowing naturally sales opportunities to better code composition and fewer bugs.
When debugging a concern, writing a failing take a look at that reproduces the bug may be a strong starting point. After the exam fails constantly, you may concentrate on repairing the bug and check out your check move when The difficulty is resolved. This technique ensures that the identical bug doesn’t return Down the road.
In short, composing checks turns debugging from a aggravating guessing video game right into a structured and predictable method—encouraging you capture extra bugs, more quickly and a lot more reliably.
Acquire Breaks
When debugging a tough issue, it’s straightforward to be immersed in the situation—gazing your screen for hours, making an attempt Alternative just after Alternative. But Among the most underrated debugging applications is solely stepping absent. Taking breaks assists you reset your brain, minimize annoyance, and sometimes see The difficulty from the new point of view.
When you are way too near to the code for far too very long, cognitive exhaustion sets in. You would possibly get started overlooking noticeable faults or misreading code that you choose to wrote just several hours before. With this state, your Mind gets to be significantly less productive at dilemma-fixing. A short wander, a espresso split, and even switching to a special job for 10–quarter-hour can refresh your concentration. A lot of developers report discovering the root of a dilemma once they've taken time for you to disconnect, letting their subconscious function in the track record.
Breaks also help avoid burnout, Particularly during extended debugging periods. Sitting before a display, mentally trapped, is not merely unproductive but also draining. Stepping absent lets you return with renewed Power and a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or a misplaced variable that eluded you ahead of.
In the event you’re trapped, an excellent general guideline is usually to set a timer—debug actively for 45–sixty minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a possibility to mature as being a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can train you a little something beneficial should you make the effort to replicate and analyze what went Incorrect.
Commence by inquiring on your own a handful of key concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with superior tactics like device tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or being familiar with and help you build stronger coding habits moving ahead.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll begin to see styles—recurring troubles or widespread blunders—which you can proactively steer clear of.
In team environments, sharing Anything you've acquired from the bug along with your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast expertise-sharing session, aiding others steer clear of the identical problem boosts workforce effectiveness and cultivates a more powerful learning society.
Far more importantly, viewing bugs as lessons shifts your frame of mind from stress to curiosity. In place of dreading bugs, you’ll start out appreciating them as vital elements of your enhancement journey. All things considered, a lot of the greatest builders will not be the ones who publish perfect code, but individuals who continuously study from their errors.
In the long run, Every bug you deal with adds a whole new layer to your ability established. So following time you squash a bug, have a second to mirror—you’ll come away a smarter, much more able developer because of it.
Conclusion
Improving upon your debugging abilities can take time, practice, and persistence — although the payoff is huge. It can make you a far more effective, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.